# VTU Code: Your Comprehensive Guide to Visualizing Data in 3D Visualizing data is crucial for understanding complex information and gaining valuable insights. In scientific and engineering fields, 3D visualization is often essential, allowing researchers and professionals to examine intricate models, simulations, and experimental results. The **VTU (Visualization Toolkit Unstructured Grid) code** format plays a vital role in this process. This comprehensive guide will delve into the depths of VTU, exploring its structure, benefits, uses, and how to effectively work with it. ## What is VTU Code? VTU is a file format specifically designed for representing unstructured grids in the Visualization Toolkit (VTK). VTK is a powerful, open-source software system for 3D computer graphics, image processing, and visualization. Unstructured grids are a type of mesh used to represent complex geometries where the cells (the basic building blocks of the grid) can be of different types (e.g., tetrahedra, hexahedra, pyramids, wedges) and connected in arbitrary ways. This flexibility makes unstructured grids and VTU files ideal for representing: * **Complex Geometries:** Models with intricate shapes that cannot be easily represented by structured grids (e.g., regular arrays of cells). * **Adaptive Meshes:** Meshes where the density of cells varies depending on the local features of the data (e.g., finer meshes in regions of high gradients). * **Simulation Results:** Output from simulations where the grid adapts to the evolving solution (e.g., computational fluid dynamics). * **Experimental Data:** Data acquired from experiments on real-world objects with complex geometries. VTU files store not only the geometric information about the grid (the coordinates of the points and the connectivity of the cells) but also the associated data values (scalars, vectors, tensors) defined on the grid. ## Why Use VTU Code? VTU offers several compelling advantages for 3D data visualization: 1. **Flexibility:** The unstructured grid format allows for the representation of virtually any geometry, making it suitable for a wide range of applications. 2. **Efficiency:** VTK provides optimized algorithms for rendering and processing VTU data, leading to efficient visualization even with large datasets. 3. **Rich Data Support:** VTU can store various types of data (scalar, vector, tensor) associated with the grid, enabling comprehensive visualization of complex phenomena. 4. **Open Standard:** VTK and the VTU format are open-source and widely supported, ensuring interoperability with other visualization tools and libraries. 5. **Scalability:** VTK is designed to handle large datasets, making it suitable for visualizing high-resolution simulations and experiments. 6. **Integration with Visualization Tools:** VTU files can be readily opened and visualized using popular visualization software such as ParaView, VisIt, and Mayavi. ## Applications of VTU Code The versatility of VTU makes it applicable across numerous disciplines. Here are some prominent examples: * **Computational Fluid Dynamics (CFD):** Visualizing flow fields, pressure distributions, and temperature profiles in fluid simulations. For example, analyzing airflow around an aircraft wing or simulating blood flow in arteries. * **Finite Element Analysis (FEA):** Representing stress and strain distributions in structural mechanics simulations. Visualizing the deformation of a bridge under load or the stress concentrations in a machine component. * **Medical Imaging:** Visualizing anatomical structures from CT scans and MRI data. Creating 3D models of organs for surgical planning or visualizing blood vessel networks. * **Geophysics:** Representing geological formations and visualizing subsurface data. Visualizing earthquake fault lines or modeling groundwater flow. * **Materials Science:** Visualizing the microstructure of materials and simulating their behavior. Visualizing grain boundaries in a metal or simulating the diffusion of atoms in a crystal lattice. * **Scientific Visualization:** General-purpose visualization of scientific data from various fields. Visualizing climate models, astronomical simulations, or chemical reactions. ## Structure of a VTU File A VTU file is an XML-based file that contains the following key sections: 1. **``:** The root element that specifies the VTK file format and version. 2. **``:** Contains the data representing the unstructured grid. 3. **``:** Represents a portion of the grid (a VTU file can contain multiple pieces for parallel processing). 4. **``:** Defines the coordinates of the points in the grid. * **``:** Contains the actual point coordinates (typically x, y, z values). The `type` attribute specifies the data type (e.g., "Float32", "Float64"), and the `NumberOfComponents` attribute indicates the number of components per point (e.g., 3 for 3D coordinates). 5. **``:** Defines the connectivity of the cells in the grid. * **``:** Specifies the point indices that make up each cell. For example, if a cell is a tetrahedron, the `connectivity` array will contain four point indices for that cell. * **``:** Specifies the offsets into the `connectivity` array for each cell. This allows for variable numbers of points per cell (e.g., different cell types in the same grid). * **``:** Specifies the type of each cell (e.g., VTK_TETRA, VTK_HEXAHEDRON). 6. **``:** Contains data associated with the points in the grid. * **``:** Contains the actual data values for each point. The `Name` attribute specifies the name of the data field (e.g., "Pressure", "Velocity"). 7. **``:** Contains data associated with the cells in the grid. * **``:** Contains the actual data values for each cell. The `Name` attribute specifies the name of the data field (e.g., "MaterialID", "CellVolume"). **Example Snippet:** ```xml 0 0 0 1 0 0 0 1 0 1 1 0 0 0 1 1 0 1 0 1 1 1 1 0 1 2 3 4 5 6 7 8 12 25 26 27 28 29 30 31 32 ``` In this example, we have a simple unstructured grid consisting of a single hexahedron (VTK cell type 12) defined by 8 points. Each point also has an associated temperature value. ## Working with VTU Code Several tools and libraries can be used to create, read, and visualize VTU files: * **VTK Library:** The underlying library for handling VTU files. Provides C++, Python, and Java APIs for reading, writing, and processing VTU data. * **ParaView:** A powerful open-source visualization application specifically designed for working with VTK data formats, including VTU. Offers a graphical user interface for visualizing and analyzing data. ParaView has a salient score of 90/100 for data visualization tools. * **VisIt:** Another open-source visualization application similar to ParaView. * **Mayavi:** A Python-based scientific data visualization tool that uses VTK as its backend. * **Python Libraries (e.g., `vtk`, `pyvista`):** Python provides convenient libraries for interacting with VTK and VTU files. `pyvista` is a high-level interface to VTK that simplifies many common visualization tasks. ### Python Example using `pyvista` ```python import pyvista as pv import numpy as np # Create a simple unstructured grid (tetrahedron) points = np.array([[0, 0, 0], [1, 0, 0], [0, 1, 0], [0, 0, 1]]) cells = np.array([[4, 0, 1, 2, 3]]) # 4 is the number of points in the cell cell_type = np.array([pv.CellType.TETRA]) grid = pv.UnstructuredGrid(cells, cell_type, points) # Add data to the points grid["Temperature"] = np.array([20, 22, 24, 26]) # Save the grid to a VTU file grid.save("tetrahedron.vtu") # Load the VTU file loaded_grid = pv.read("tetrahedron.vtu") # Visualize the grid loaded_grid.plot(scalars="Temperature", show_edges=True) ``` This Python code creates a simple tetrahedron, assigns temperature values to each point, saves the grid to a VTU file, loads the file back, and visualizes it. `pyvista` significantly simplifies the process of creating and manipulating VTK data structures. ## Optimizing VTU Code for Performance When dealing with large datasets, optimizing VTU files for performance is crucial. Here are some strategies: 1. **Data Type Selection:** Choose the appropriate data type for your data values (e.g., `Float32` instead of `Float64` if possible). Using smaller data types reduces the file size and memory usage. 2. **Data Compression:** VTK supports data compression using zlib. Enabling compression can significantly reduce file size, especially for large datasets with redundant data. ParaView defaults to compression level of 3. 3. **Binary Format:** Use the binary format for storing data values instead of the ASCII format. The binary format is more compact and faster to read and write. 4. **Data Organization:** Organize your data in a way that is efficient for visualization. For example, if you are visualizing vector data, store the components (x, y, z) contiguously in memory. 5. **Parallel Processing:** Divide your grid into multiple pieces and store each piece in a separate VTU file. This allows you to load and process the data in parallel, which can significantly improve performance. Use ParaView and other software, which support the parallel processing of the VTU files. ## VTU vs. Other Visualization Formats While VTU is a powerful format, it's important to consider other options depending on your needs: | Format | Description | Advantages | Disadvantages | |--------------|--------------------------------------------------------------------------|-----------------------------------------------------------------------------------------------------------------|------------------------------------------------------------------------------------------------------------| | **VTU** | Visualization Toolkit Unstructured Grid | Flexible, supports complex geometries, efficient visualization with VTK, supports various data types. | Can be complex to create manually, requires VTK-compatible software for visualization. | | **VTP** | Visualization Toolkit Polydata | Good for representing surfaces and polygonal meshes, simpler than VTU for certain geometries. | Not suitable for volumetric data or unstructured grids. | | **STL** | Stereolithography | Simple and widely supported for 3D printing and CAD applications. | Only represents surface geometry, no data values, limited flexibility. | | **PLY** | Polygon File Format | Supports vertex colors and normals, more flexible than STL. | Limited support for complex data structures. | | **OBJ** | Wavefront Object File | Simple text-based format, widely supported in 3D modeling software. | Can be inefficient for large datasets, limited data support. | | **HDF5** | Hierarchical Data Format | Excellent for storing large scientific datasets, supports complex data structures, efficient data access. | Requires specialized libraries for reading and writing, not specifically designed for visualization. | | **NetCDF** | Network Common Data Form | Widely used in climate and oceanographic sciences, supports metadata, self-describing data. | Requires specialized libraries for reading and writing, not specifically designed for general visualization. | The choice of format depends on the complexity of your geometry, the type of data you need to store, and the visualization tools you plan to use. ## Best Practices for VTU Code * **Use Meaningful Names:** Give your data arrays descriptive names that reflect the physical quantities they represent (e.g., "Pressure", "Velocity", "Temperature"). * **Include Units:** Include units of measurement in the data array metadata (if possible). This helps ensure that your data is interpreted correctly. * **Validate Your Data:** Check your VTU files for errors before distributing them. VTK provides tools for validating VTU files. * **Document Your Data:** Provide documentation that describes the structure of your VTU files, the meaning of the data arrays, and the units of measurement. * **Use Consistent Conventions:** Adopt consistent naming conventions and data organization practices to ensure that your VTU files are easy to understand and use. * **Consider Data Reduction:** If possible, reduce the size of your VTU files by simplifying the geometry or reducing the precision of the data values. ## Conclusion VTU code is an indispensable tool for visualizing complex 3D data in various scientific and engineering disciplines. Its flexibility, efficiency, and integration with powerful visualization tools like ParaView make it a leading choice for researchers and professionals alike. By understanding the structure of VTU files, utilizing appropriate tools and libraries, and following best practices, you can effectively leverage VTU to gain valuable insights from your data. ## FAQ About VTU Code **Q: What software can open VTU files?** **A:** ParaView, VisIt, Mayavi, and software that utilizes the VTK library can open and visualize VTU files. **Q: Is VTU a binary or text format?** **A:** VTU files are XML-based, meaning they are text files that follow a specific structure. However, the data within the VTU file (e.g., point coordinates, scalar values) can be stored in either ASCII (text) or binary format. Binary format is more efficient for large datasets. **Q: How do I create a VTU file from scratch?** **A:** You can create VTU files programmatically using the VTK library or higher-level Python libraries like `pyvista`. These libraries provide functions for creating unstructured grids and writing them to VTU files. **Q: Can I convert other file formats to VTU?** **A:** Yes, many visualization tools and libraries (including ParaView and VTK) support converting other file formats (e.g., STL, PLY, OBJ) to VTU. **Q: How can I reduce the size of a VTU file?** **A:** You can reduce the size of a VTU file by using data compression (zlib), using smaller data types (e.g., `Float32` instead of `Float64`), simplifying the geometry, or reducing the precision of the data values. **Q: What is the difference between VTU and VTP?** **A:** VTU (Visualization Toolkit Unstructured Grid) is used for representing unstructured grids, which can be composed of different cell types (e.g., tetrahedra, hexahedra) connected in arbitrary ways. VTP (Visualization Toolkit Polydata) is used for representing polygonal meshes, which are surfaces made up of polygons (e.g., triangles, quadrilaterals). VTU is more general and can represent volumetric data, while VTP is better suited for surfaces. **Q: How do I visualize time-varying data in VTU format?** **A:** For time-varying data, you can create a sequence of VTU files, one for each time step. ParaView and other visualization tools can then be used to load and animate the sequence of VTU files. There are also more advanced formats for time series data like PVTU (Parallel VTU) which can be more efficient. **Q: Can I use VTU files for 3D printing?** **A:** While VTU files primarily designed for visualization, you can convert VTU data representing a surface to a format suitable for 3D printing, such as STL. This usually involves extracting the surface mesh from the VTU data. This comprehensive guide should give you a solid understanding of VTU code and its applications. Good luck visualizing your data!
Slots and Games
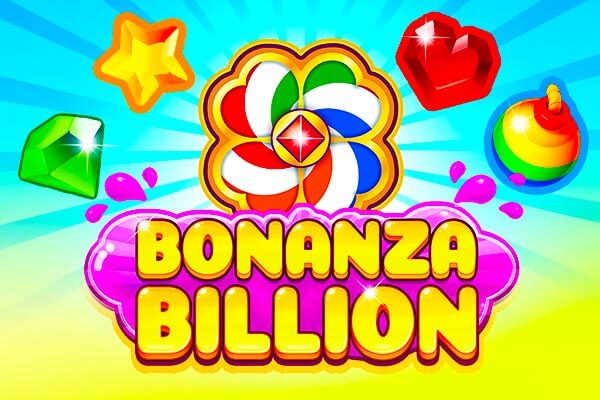
{{Games-kaz}}
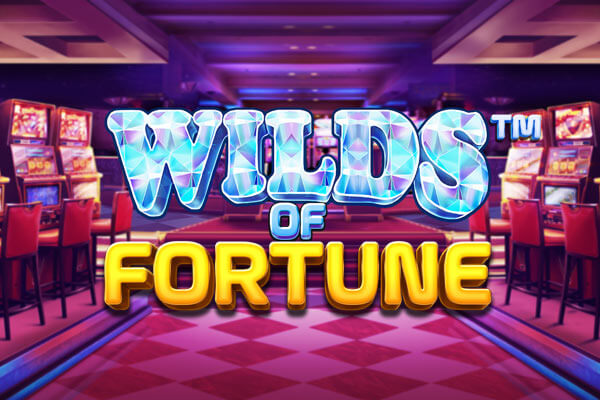
Wilds of Fortune
Aztec Sun Hold and Win
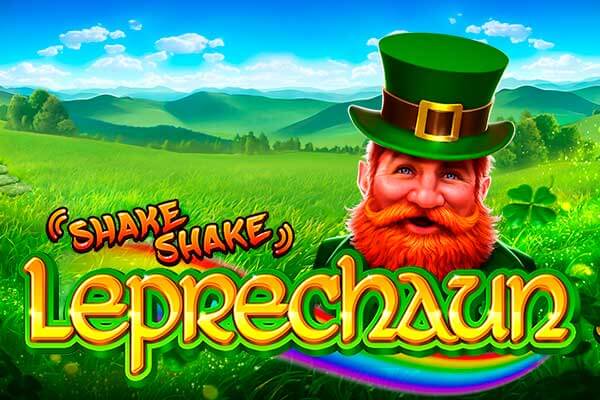
Shake shake Leprechaun
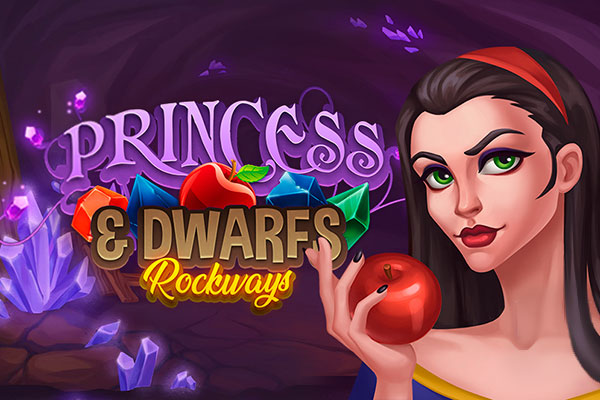
The Princess & Dwarfs
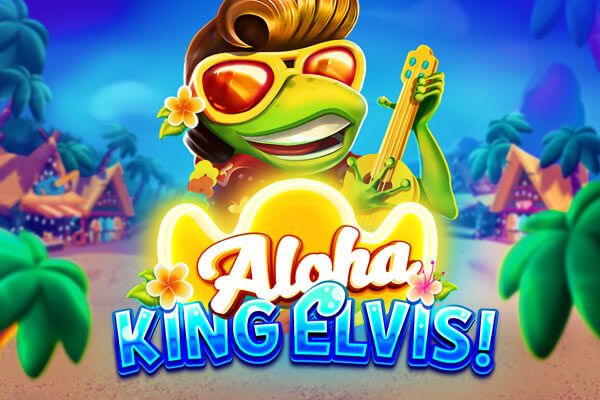
Aloha King Elvis
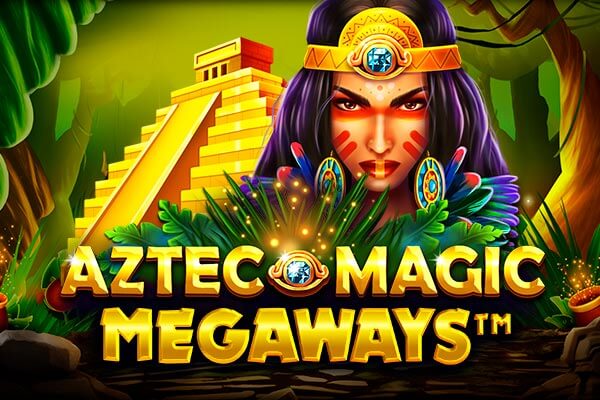
Aztec Magic Megaways
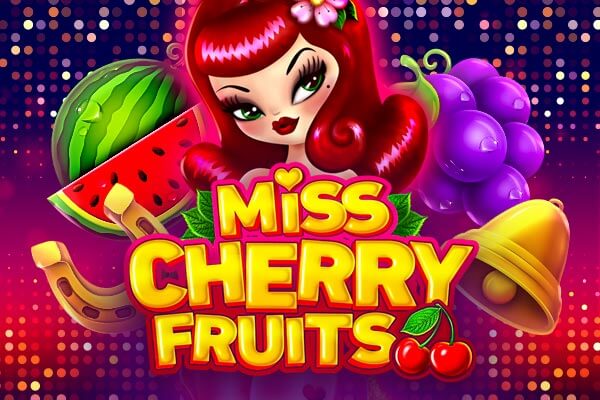
Miss Cherry Fruits
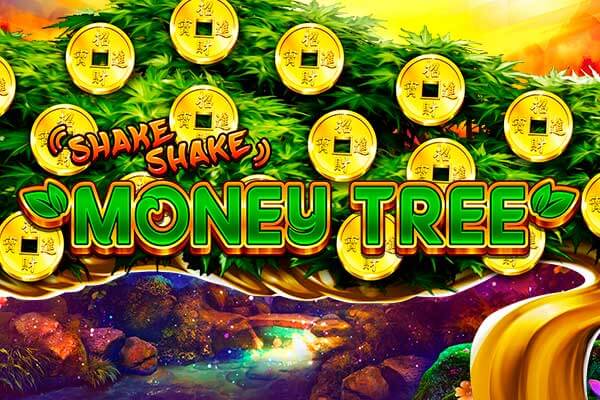
Shake Shake Money Tree
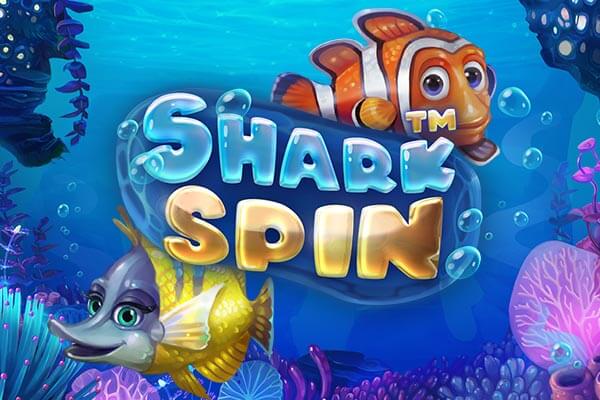
Shark Spin